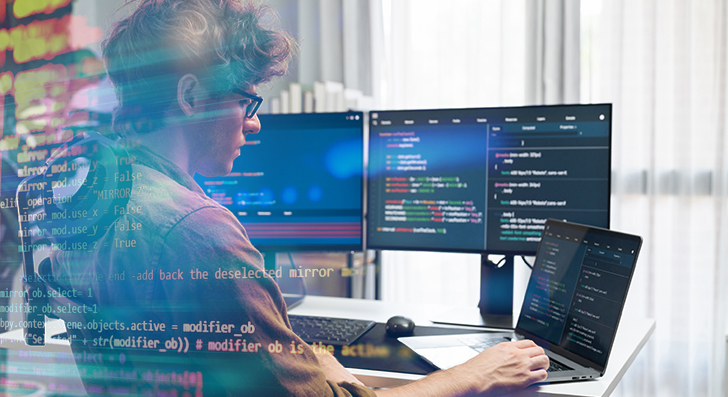
Scalability usually means your software can handle advancement—additional consumers, much more details, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and sensible tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be portion of your system from the beginning. Quite a few applications fall short once they improve quick because the initial design can’t take care of the additional load. Like a developer, you might want to Feel early regarding how your method will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases in which anything is tightly connected. As an alternative, use modular structure or microservices. These designs crack your application into smaller sized, impartial pieces. Every module or provider can scale By itself without impacting The complete system.
Also, take into consideration your databases from working day one. Will it require to deal with 1,000,000 people or perhaps 100? Select the suitable type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t need them nevertheless.
A different significant issue is to avoid hardcoding assumptions. Don’t create code that only performs less than present-day conditions. Consider what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style designs that help scaling, like message queues or celebration-pushed programs. These support your app manage a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you're not just preparing for success—you might be cutting down long run complications. A effectively-planned system is less complicated to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Database
Deciding on the appropriate database is a vital part of setting up scalable apps. Not all databases are developed the same, and utilizing the Improper one can gradual you down and even cause failures as your app grows.
Start out by comprehension your information. Can it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective fit. They are solid with relationships, transactions, and regularity. They also aid scaling tactics like read replicas, indexing, and partitioning to manage more website traffic and information.
In case your facts is more versatile—like user action logs, products catalogs, or documents—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling significant volumes of unstructured or semi-structured info and will scale horizontally much more simply.
Also, consider your go through and generate patterns. Do you think you're doing a lot of reads with fewer writes? Use caching and read replicas. Will you be managing a hefty publish load? Take a look at databases that may take care of significant write throughput, and even celebration-based info storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You might not have to have Sophisticated scaling functions now, but picking a databases that supports them means you won’t require to change later on.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts based upon your obtain patterns. And often check database functionality while you expand.
In a nutshell, the best database is determined by your app’s construction, speed demands, And the way you assume it to expand. Get time to pick wisely—it’ll save a lot of hassle afterwards.
Enhance Code and Queries
Quickly code is essential to scalability. As your application grows, every single tiny delay provides up. Improperly published code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to Establish efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward a person will work. Keep your capabilities limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also lengthy to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These usually gradual items down much more than the code by itself. Make sure Every single query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, especially across substantial tables.
In the event you observe the same info staying check here requested repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred documents might crash after they have to deal with one million.
Briefly, scalable applications are rapid applications. Keep the code tight, your queries lean, and use caching when required. These measures support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more people plus much more website traffic. If every thing goes via 1 server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. Both of these applications enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming traffic across multiple servers. Instead of one server accomplishing many of the get the job done, the load balancer routes end users to distinct servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the others. Instruments like Nginx, HAProxy, or cloud-based mostly answers from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for precisely the same details again—like an item web page or perhaps a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two frequent types of caching:
one. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t alter often. And constantly make sure your cache is up to date when details does modify.
Briefly, load balancing and caching are easy but strong tools. Collectively, they assist your application deal with far more buyers, remain rapidly, and Recuperate from troubles. If you propose to develop, you may need both of those.
Use Cloud and Container Tools
To create scalable apps, you would like tools that allow your app improve conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to rent servers and solutions as you will need them. You don’t really need to get components or guess long run ability. When targeted visitors improves, you can add a lot more assets with only a few clicks or routinely employing car-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to developing your app instead of running infrastructure.
Containers are An additional key Software. A container offers your app and every little thing it must operate—code, libraries, configurations—into one particular unit. This can make it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also ensure it is easy to individual elements of your application into companies. You are able to update or scale sections independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get better rapidly when challenges occur. If you prefer your app to improve without boundaries, start making use of these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Keep an eye on All the things
In the event you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is undertaking, spot problems early, and make improved decisions as your app grows. It’s a essential Element of building scalable methods.
Start off by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your application far too. Keep an eye on how long it takes for customers to load webpages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or maybe a assistance goes down, it is best to get notified promptly. This will help you take care of difficulties rapidly, typically just before customers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes serious problems.
As your app grows, traffic and facts boost. With out monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the right instruments in place, you keep in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Ideas
Scalability isn’t only for huge providers. Even tiny apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that improve smoothly with no breaking stressed. Start modest, Imagine large, and Create smart.